代理模式 - 动态代理
2024-09-23 16:39 由
读书声起五更天 发表于
#后端开发
动态代理的API
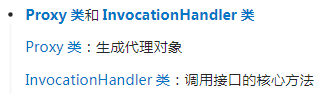
Proxy 动态代理类
- 生成代理对象:Proxy.newProxyInstance( 类加载器,接口数组,处理器 )
- 类加载器:对象.getClass( ).getClassLoader( )
- 接口数组-被代理类的所有接口:被代理对象.getClass( ).getInterfaces( )
- 处理器:代理对象调用方法时,会被处理器拦截
InvocationHandler 处理器接口
- 处理器的作用:拦截方法的执行
- 代理对象调用方法时,必须经过处理器的invoke( )方法
动态代理的角色分析
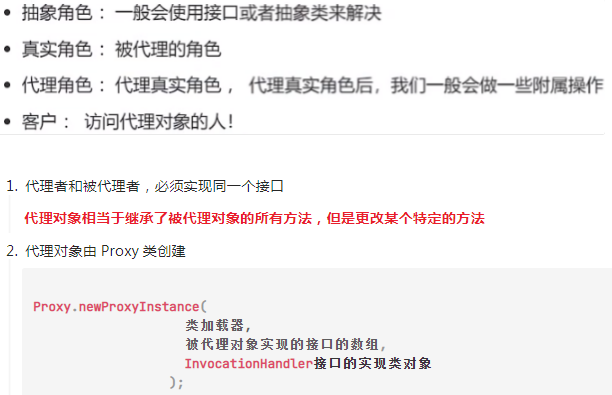
动态代理的模板代码
// 动态代理公式
// T t=(T)Proxy.newProxyInstance(类加载器,接口数组,处理器);
T t=(T)Proxy.newProxyInstance(
被代理对象.getClass().getClassLoader(),
被代理对象.getClass().getInterfaces();
(Object obj, Method method, Object[] args)->{
//方法执行前
Object result = method.invoke(代理对象,args);
//方法执行后
return result;
}
);
t.接口的方法() ;
应用实例
调用List集合remove( )时,删除指定的元素(包含重复的元素)
public static void main(String[] args1) {
//需求:调用List集合remove()时,删除指定的元素,包含多个重复的元素
//1.创建被代理对象
List list = new ArrayList();
Collections.addAll(list, 2, 3, 5, 2, 7, 2, 9,5, 6);
System.out.println("调用方法前:"+list);
//2.通过Proxy类 创建代理对象
List listProxy=(List) Proxy.newProxyInstance(
list.getClass().getClassLoader(),
list.getClass().getInterfaces(),
(Object obj, Method method, Object[] args)->{
Object invoke = null;//目标方法的返回值,如果没有,则不需要写
if("remove".equals(method.getName())){
//调用remove方法时,删除所有指定的重复元素
while(true){
if (!list.contains(args[0])) {
break;
}
list.remove(args[0]);
}
}else{
//其它方法,正常执行
invoke = method.invoke(list, args);
}
return invoke;
}
);
//3.代理对象调用方法
listProxy.remove(2);
System.out.println("调用方法后:"+list);
}